Calling APIs from the bot using the code template
The code template in conditional responses, and the code snippet response type allows developers to configure bot responses using Python code. Even though code in responses can be used for a variety of operations, the central purpose is to make API calls in cases where bot responses need to be dynamic and rely on information from external systems.
The simplest and most elegant way of using HTTP APIs with Python is using the Requests library.
In this article, we will look at an example where we make a GET request and use certain values from the API response to formulate bot response.
The user’s intent in this example is to check the weather. On detecting the intent, bot prompts the user for a city name. The response template is configured to make an API request once the user provides a valid city name. The code for this will look like:
import requests
#Get the value of city provided by the user from entities
city = variables['newdfState']['model_state']['entities']['loc']['value']
url = "http://api.weatherstack.com/current"
payload={'access_key':'your-api-access_key', 'query':city}
headers = {'Content-Type':'application/json'}
#API request
response = requests.request("GET", url, headers=headers, params=payload)
#Extracting the details we want to use in bot response from the API response
temp=response.json()['current']['temperature']
feel=response.json()['current']['feelslike']
#Storing the above values in data store
variables['dataStore']['feel']=feel
variables['dataStore']['temp']=temp
#Updating the data store
output = {
"dataStore": variables['dataStore']
}
The data store variables defined above can be used in bot response:
“The current temperature in ${newdfState.model_state.entities.loc.value} is ${dataStore.temp}°C, feels like ${dataStore.feel}°C.”
The response content in bot builder:
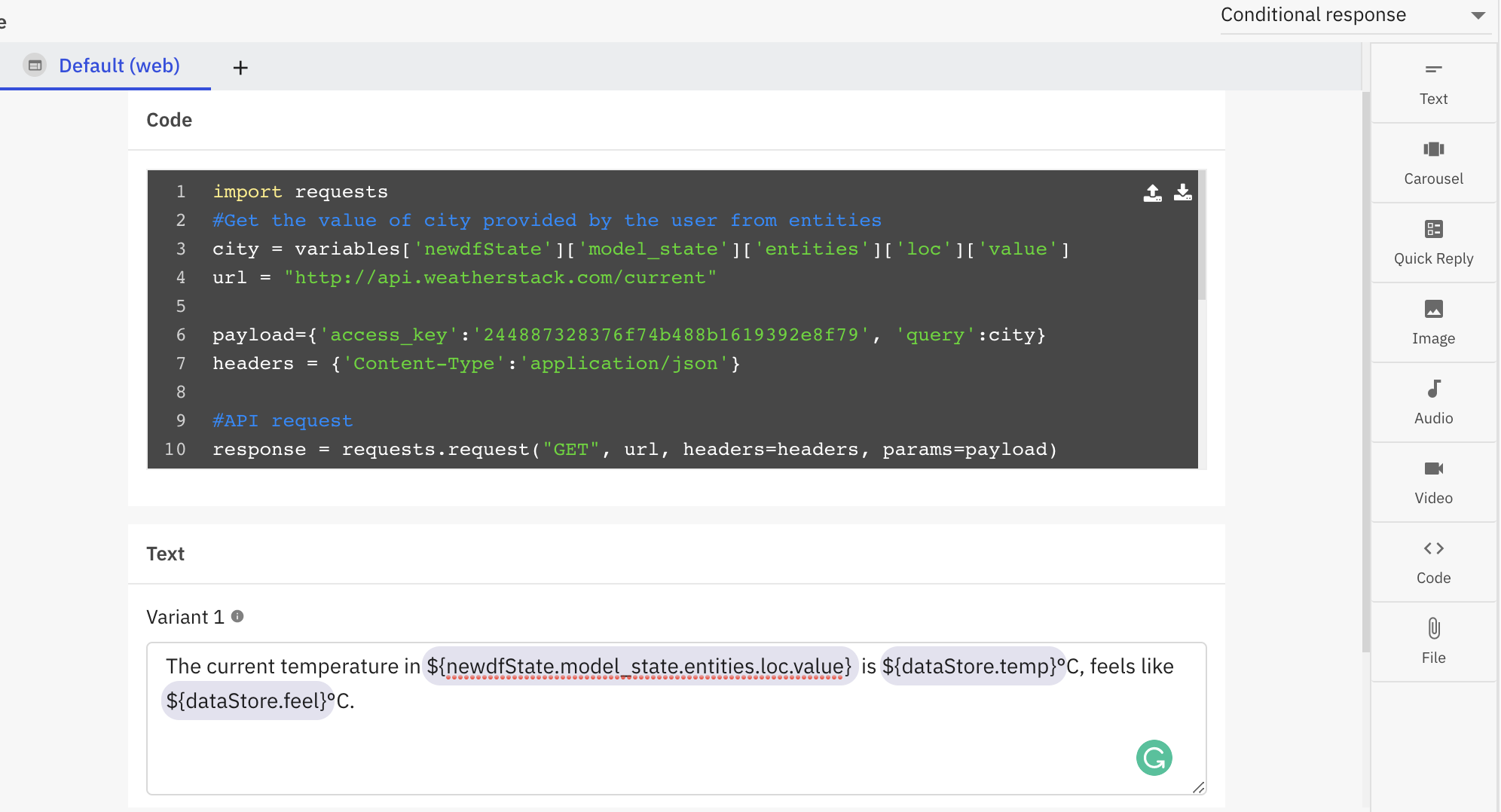
Make API calls from the bot
The same outcome can be achieved by using code snippet in place of conditional response designer. The code in this case will be:
import requests
#Get the value of city provided by the user from entities
city = variables['newdfState']['model_state']['entities']['loc']['value']
url = "http://api.weatherstack.com/current"
payload={'access_key':'244887328376f74b488b1619392e8f79', 'query':city}
headers = {'Content-Type':'application/json'}
#API request
response = requests.request("GET", url, headers=headers, params=payload)
#Extracting the details we want to use in bot response from the API response
temp=response.json()['current']['temperature']
feel=response.json()['current']['feelslike']
#Using the above variables in bot response
output = {
"generated_msg": [
{"text": ["The current temperature in "+city+" is " +str(temp)+"°C, feels like "+str(feel)+"°C."]}
]
}
he major difference here is constructing the bot response using code snippet instead of storing variables in data store and using them in text template.
Updated 5 months ago